Note
Go to the end to download the full example code.
LS-Dyna analysis#
Using supplied files, this example shows how to insert an LS-Dyna analysis into a new Mechanical session and execute a sequence of Python scripting commands that define and solve the analysis. Deformation results are then reported and plastic strain (EPS) animation is exported in the project directory.
Download required files#
Download the required files. Print the file path for the geometry file.
import os
from PIL import Image
from ansys.mechanical.core import launch_mechanical
from ansys.mechanical.core.examples import download_file
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
from matplotlib.animation import FuncAnimation
geometry_path = download_file("example_08_Taylor_Bar.agdb", "pymechanical", "00_basic")
print(f"Downloaded the geometry file to: {geometry_path}")
Downloaded the geometry file to: /home/runner/.local/share/ansys_mechanical_core/examples/example_08_Taylor_Bar.agdb
Launch mechanical#
Launch a new Mechanical session in batch, setting cleanup_on_exit
to
False
. To close this Mechanical session when finished, this example
must call the mechanical.exit()
method.
mechanical = launch_mechanical(batch=True, cleanup_on_exit=False)
print(mechanical)
Ansys Mechanical [Ansys Mechanical Enterprise]
Product Version:242
Software build date: 06/03/2024 09:35:09
Initialize variable for workflow#
Set the part_file_path
variable on the server for later use.
Make this variable compatible for Windows, Linux, and Docker containers.
project_directory = mechanical.project_directory
print(f"project directory = {project_directory}")
project_directory = project_directory.replace("\\", "\\\\")
mechanical.run_python_script(f"project_directory='{project_directory}'")
# Upload the file to the project directory.
mechanical.upload(file_name=geometry_path, file_location_destination=project_directory)
# Build the path relative to project directory.
base_name = os.path.basename(geometry_path)
combined_path = os.path.join(project_directory, base_name)
part_file_path = combined_path.replace("\\", "\\\\")
mechanical.run_python_script(f"part_file_path='{part_file_path}'")
project directory = /tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/
Uploading example_08_Taylor_Bar.agdb to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/.: 0%| | 0.00/2.27M [00:00<?, ?B/s]
Uploading example_08_Taylor_Bar.agdb to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/.: 100%|██████████| 2.27M/2.27M [00:00<00:00, 277MB/s]
''
Download required material files#
Download the required file. Print the file path for the material file.
mat_st_path = download_file("example_08_Taylor_Bar_Mat.xml", "pymechanical", "00_basic")
print(f"Downloaded the material file to: {mat_st_path}")
# Upload the file to the project directory.
mechanical.upload(file_name=mat_st_path, file_location_destination=project_directory)
# Build the path relative to project directory.
base_name = os.path.basename(mat_st_path)
combined_path = os.path.join(project_directory, base_name)
mat_file_path = combined_path.replace("\\", "\\\\")
mechanical.run_python_script(f"mat_file_path='{mat_file_path}'")
# Verify the path
result = mechanical.run_python_script("part_file_path")
print(f"part_file_path on server: {result}")
Downloaded the material file to: /home/runner/.local/share/ansys_mechanical_core/examples/example_08_Taylor_Bar_Mat.xml
Uploading example_08_Taylor_Bar_Mat.xml to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/.: 0%| | 0.00/13.6k [00:00<?, ?B/s]
Uploading example_08_Taylor_Bar_Mat.xml to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/.: 100%|██████████| 13.6k/13.6k [00:00<00:00, 44.2MB/s]
part_file_path on server: /tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/example_08_Taylor_Bar.agdb
Run the script#
Run the Mechanical script to attach the geometry and set up and solve the analysis.
mech_act_code = """
import os
import json
# Import Taylor bar geometry
geometry_import_group = Model.GeometryImportGroup
geometry_import = geometry_import_group.AddGeometryImport()
geometry_import_format = Ansys.Mechanical.DataModel.Enums.GeometryImportPreference.Format.Automatic
geometry_import.Import(part_file_path, geometry_import_format, None)
Model.AddLSDynaAnalysis()
analysis = Model.Analyses[0]
ExtAPI.Application.ActiveUnitSystem = MechanicalUnitSystem.StandardNMMton
ExtAPI.Application.ActiveAngleUnit = AngleUnitType.Radian
# Assign the material
MAT = ExtAPI.DataModel.Project.Model.Materials
MAT.Import(mat_file_path)
ExtAPI.DataModel.Project.Model.Geometry.Children[0].Children[0].Material = "Bullet"
# Add Coordinate system
cs = Model.CoordinateSystems
lcs = cs.AddCoordinateSystem()
lcs.Origin = [10.0, 1.5, -10.0]
lcs.PrimaryAxis = CoordinateSystemAxisType.PositiveZAxis
lcs.PrimaryAxisDefineBy = CoordinateSystemAlignmentType.GlobalY
lcs.OriginDefineBy = CoordinateSystemAlignmentType.Fixed
solver = analysis.Solver
solver.Properties['Step Controls/Endtime'].Value = 3.0E-5
analysis.Activate()
# Add Rigid Wall
rigid_wall = analysis.CreateLoadObject("Rigid Wall", "LSDYNA")
rigid_wall.Properties["Coordinate System"].Value = lcs.ObjectId
ExtAPI.DataModel.Tree.Refresh()
# Adding initial velocity
ic = ExtAPI.DataModel.GetObjectsByName("Initial Conditions")[0]
vel = ic.InsertVelocity()
selection = ExtAPI.SelectionManager.CreateSelectionInfo(SelectionTypeEnum.GeometryEntities)
selection.Ids = [ExtAPI.DataModel.GeoData.Assemblies[0].Parts[0].Bodies[0].Id]
vel.Location = selection
vel.DefineBy = LoadDefineBy.Components
vel.YComponent = Quantity(-280000, ExtAPI.DataModel.CurrentUnitFromQuantityName("Velocity"))
# By default quadratic element order in Mechanical - LSDyna supports only Linear
mesh = ExtAPI.DataModel.GetObjectsByName("Mesh")[0]
mesh.ElementOrder = ElementOrder.Linear
mesh.ElementSize = Quantity(0.5, "mm")
# Solve
analysis.Solution.Solve()
# Post-processing
eps = analysis.Solution.AddUserDefinedResult()
eps.Expression = "EPS"
eps.EvaluateAllResults()
eps_max = eps.Maximum
eps_min = eps.Minimum
total_deformation = analysis.Solution.AddTotalDeformation()
total_deformation.EvaluateAllResults()
# Set Camera
Graphics.Camera.FocalPoint = Point([9.0521184381880495,
2.9680547361873595,
-11.52925245328758], 'mm')
Graphics.Camera.ViewVector = Vector3D(0.5358281613965048,
-0.45245539014067604,
0.71286204933850261)
Graphics.Camera.UpVector = Vector3D(-0.59927496479653264,
0.39095266724498329,
0.69858823962485084)
Graphics.Camera.SceneHeight = Quantity(14.66592829617538, 'mm')
Graphics.Camera.SceneWidth = Quantity(8.4673776497126063, 'mm')
# Set Scale factor
true_scale = MechanicalEnums.Graphics.DeformationScaling.True
Graphics.ViewOptions.ResultPreference.DeformationScaling = true_scale
Graphics.ViewOptions.ResultPreference.DeformationScaleMultiplier = 1
# Export an animation
mechdir = ExtAPI.DataModel.AnalysisList[0].WorkingDir
eps.Activate()
animation_export_format = GraphicsAnimationExportFormat.GIF
settings_720p = Ansys.Mechanical.Graphics.AnimationExportSettings()
settings_720p.Width = 1280
settings_720p.Height = 720
anim_file_path = os.path.join(mechdir, "taylor_bar.gif")
eps.ExportAnimation(
anim_file_path, animation_export_format, settings_720p
)
# Set the isometric view and zoom to fit
settings_720p = Ansys.Mechanical.Graphics.GraphicsImageExportSettings()
settings_720p.Resolution = (GraphicsResolutionType.EnhancedResolution)
settings_720p.Background = GraphicsBackgroundType.White
settings_720p.Width = 1280
settings_720p.Height = 720
settings_720p.CurrentGraphicsDisplay = False
total_deformation.Activate()
image_path = os.path.join(mechdir, "totaldeformation.png")
Graphics.ExportImage(image_path, GraphicsImageExportFormat.PNG, settings_720p)
dir_deformation_details = {
"Minimum": str(eps_max),
"Maximum": str(eps_min)
}
json.dumps(dir_deformation_details)
"""
output = mechanical.run_python_script(mech_act_code)
print(output)
# image_directory_modified = project_directory.replace("\\", "\\\\")
mechanical.run_python_script(f"image_dir=ExtAPI.DataModel.AnalysisList[0].WorkingDir")
# Verify the path for image directory.
result_image_dir_server = mechanical.run_python_script(f"image_dir")
print(f"Images are stored on the server at: {result_image_dir_server}")
{"Maximum": "0", "Minimum": "2.7366569995880128"}
Images are stored on the server at: /tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/
Download output file from solve and print contents#
Download the solve.out
file from the server to the current working
directory and print the contents. Remove the solve.out
file.
def get_solve_out_path(mechanical):
"""Get the solve out path and return."""
solve_out_path = ""
for file_path in mechanical.list_files():
if file_path.find("solve.out") != -1:
solve_out_path = file_path
break
return solve_out_path
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
print("Getting solve out path")
solve_out_path = get_solve_out_path(mechanical)
print(f"Solve out path (on remote machine): {solve_out_path}")
if solve_out_path != "":
current_working_directory = os.getcwd()
print(f"Performing download request...")
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
print(f"File downloaded locally at {solve_out_local_path}")
write_file_contents_to_console(solve_out_local_path)
print("Printed output to console")
os.remove(solve_out_local_path)
Getting solve out path
Solve out path (on remote machine): /tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/solve.out
Performing download request...
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/solve.out to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/solve.out: 0%| | 0.00/13.7k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/solve.out to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/solve.out: 100%|██████████| 13.7k/13.7k [00:00<00:00, 66.6MB/s]
File downloaded locally at /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/solve.out
License option : check ansys licenses only
***************************************************************
* ANSYS LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2023 ANSYS, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of ANSYS, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the ANSYS, Inc. online documentation or the ANSYS, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* ANSYS, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* ANSYS, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the ANSYS, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the ANSYS, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
Date: 11/04/2024 Time: 14:50:50
___________________________________________________
| |
| LS-DYNA, A Program for Nonlinear Dynamic |
| Analysis of Structures in Three Dimensions |
| Date : 03/26/2024 Time: 16:37:49 |
| Version : smp s R14 |
| Revision: R14.1.1-4-gaf1eb871e8 |
| AnLicVer: 2024 R2 (20240501+dl-65-gb272478) |
| |
| Features enabled in this version: |
| Shared Memory Parallel |
| FFTW (multi-dimensional FFTW Library) |
| ANSYSLIC enabled |
| |
| Platform : Xeon64 System |
| OS Level : Linux 3.10.0 uum |
| Compiler : Intel Fortran Compiler 19.0 SSE2 |
| Hostname : 938793e4e167 |
| Precision : Single precision (I4R4) |
| |
| Unauthorized use infringes Ansys Inc. copyrights |
|___________________________________________________|
> i=input.k S=input.intfor x=70 NCPU=1
[license/info] Successfully checked out 1 of "dyna_solver_core".
[license/info] --> Checkout ID: 938793e4e167-root-122-000157 (days left: 169)
[license/info] --> Customer ID: 0
[license/info] Successfully started "LSDYNA (Core-based License)".
Executing with ANSYS license
Scale factor for file size = 70
new file size = 18350080
Use this scale factor on all restarts.
Command line options: i=input.k
S=input.intfor
x=70
NCPU=1
Input file: input.k
The native file format : 32-bit small endian
Memory size from default : 100000000
on UNIX computers note the following change:
ctrl-c interrupts ls-dyna and prompts for a sense switch.
type the desired sense switch: sw1., sw2., etc. to continue
the execution. ls-dyna will respond as explained in the users manual
type response
----- ------------------------------------------------------------
quit ls-dyna terminates.
stop ls-dyna terminates.
sw1. a restart file is written and ls-dyna terminates.
sw2. ls-dyna responds with time and cycle numbers.
sw3. a restart file is written and ls-dyna continues calculations.
sw4. a plot state is written and ls-dyna continues calculations.
sw5. ls-dyna enters interactive graphics phase.
swa. ls-dyna flushes all output i/o buffers.
swb. a dynain is written and ls-dyna continues calculations.
swc. a restart and dynain are written and ls-dyna continues calculations.
swd. a restart and dynain are written and ls-dyna terminates.
swe. stop dynamic relaxation just as though convergence
endtime=time change the termination time
lpri toggle implicit lin. alg. solver output on/off.
nlpr toggle implicit nonlinear solver output on/off.
iter toggle implicit output to d3iter database on/off.
prof output timing data to messag and continue.
conv force implicit nonlinear convergence for current time step.
ttrm terminate implicit time step, reduce time step, retry time step.
rtrm terminate implicit at end of current time step.
******** notice ******** notice ******** notice ********
* *
* This is the LS-DYNA Finite Element code. *
* *
* Neither LST nor the authors assume any responsibility for *
* the validity, accuracy, or applicability of any results *
* obtained from this system. Users must verify their own *
* results. *
* *
* LST endeavors to make the LS-DYNA code as complete, *
* accurate and easy to use as possible. *
* Suggestions and comments are welcomed. Please report any *
* errors encountered in either the documentation or results *
* immediately to LST through your site focus. *
* *
* Copyright (C) 1990-2021 *
* by Livermore Software Technology, LLC *
* All rights reserved *
* *
******** notice ******** notice ******** notice ********
Beginning of keyword reader 11/04/24 14:50:51
Memory required to process keyword : 319296
Additional dynamic memory required : 573821
input of data is completed
initial kinetic energy = 0.68292406E+05
Memory required to begin solution : 320K
Additional dynamically allocated memory: 787K
Total: 1107K
initialization completed
1 t 0.0000E+00 dt 1.98E-08 flush i/o buffers 11/04/24 14:50:51
1 t 0.0000E+00 dt 1.98E-08 write d3plot file 11/04/24 14:50:51
cpu time per zone cycle............ 383 nanoseconds
average cpu time per zone cycle.... 415 nanoseconds
average clock time per zone cycle.. 473 nanoseconds
estimated total cpu time = 3 sec ( 0 hrs 0 mins)
estimated cpu time to complete = 3 sec ( 0 hrs 0 mins)
estimated total clock time = 3 sec ( 0 hrs 0 mins)
estimated clock time to complete = 3 sec ( 0 hrs 0 mins)
termination time = 3.000E-05
termination cycle = 10000000
77 t 1.4949E-06 dt 1.98E-08 write d3plot file 11/04/24 14:50:51
153 t 2.9937E-06 dt 1.97E-08 write d3plot file 11/04/24 14:50:51
229 t 4.4954E-06 dt 1.99E-08 write d3plot file 11/04/24 14:50:51
304 t 5.9808E-06 dt 2.00E-08 write d3plot file 11/04/24 14:50:52
380 t 7.4942E-06 dt 1.98E-08 write d3plot file 11/04/24 14:50:52
461 t 8.9899E-06 dt 1.59E-08 write d3plot file 11/04/24 14:50:52
564 t 1.0494E-05 dt 1.36E-08 write d3plot file 11/04/24 14:50:52
689 t 1.1990E-05 dt 1.07E-08 write d3plot file 11/04/24 14:50:52
836 t 1.3497E-05 dt 9.66E-09 write d3plot file 11/04/24 14:50:53
1003 t 1.4998E-05 dt 8.72E-09 write d3plot file 11/04/24 14:50:53
1190 t 1.6499E-05 dt 7.94E-09 write d3plot file 11/04/24 14:50:53
1392 t 1.7994E-05 dt 7.34E-09 write d3plot file 11/04/24 14:50:53
1604 t 1.9498E-05 dt 6.63E-09 write d3plot file 11/04/24 14:50:54
1827 t 2.0996E-05 dt 6.76E-09 write d3plot file 11/04/24 14:50:54
2058 t 2.2500E-05 dt 6.30E-09 write d3plot file 11/04/24 14:50:55
2289 t 2.3999E-05 dt 6.27E-09 write d3plot file 11/04/24 14:50:55
2524 t 2.5494E-05 dt 6.30E-09 write d3plot file 11/04/24 14:50:55
2762 t 2.6996E-05 dt 6.32E-09 write d3plot file 11/04/24 14:50:56
2992 t 2.8499E-05 dt 6.71E-09 write d3plot file 11/04/24 14:50:56
3225 t 2.9998E-05 dt 6.33E-09 write d3plot file 11/04/24 14:50:56
*** termination time reached ***
3225 t 3.0004E-05 dt 6.33E-09 write d3dump01 file 11/04/24 14:50:56
3225 t 3.0004E-05 dt 6.33E-09 write d3plot file 11/04/24 14:50:56
N o r m a l t e r m i n a t i o n 11/04/24 14:50:56
Memory required to complete solution : 320K
Additional dynamically allocated memory: 789K
Total: 1108K
T i m i n g i n f o r m a t i o n
CPU(seconds) %CPU Clock(seconds) %Clock
----------------------------------------------------------------
Keyword Processing ... 2.7839E-02 0.51 2.7840E-02 0.45
KW Reading ......... 1.7579E-02 0.32 1.7580E-02 0.28
KW Writing ......... 1.9541E-03 0.04 1.9550E-03 0.03
Initialization ....... 1.0370E-01 1.89 8.7799E-01 14.04
Init Proc Phase 1 .. 8.4434E-03 0.15 9.4780E-03 0.15
Init Proc Phase 2 .. 2.4902E-03 0.05 3.1650E-03 0.05
Element processing ... 4.4943E+00 82.07 4.4942E+00 71.87
Solids ............. 4.1214E+00 75.26 4.1202E+00 65.89
Shells ............. 7.7679E-03 0.14 8.8120E-03 0.14
ISO Shells ......... 3.1011E-03 0.06 3.8500E-03 0.06
E Other ............ 9.0143E-03 0.16 9.2340E-03 0.15
Binary databases ..... 2.2056E-02 0.40 2.2538E-02 0.36
ASCII database ....... 1.4908E-01 2.72 1.4875E-01 2.38
Contact algorithm .... 8.4073E-03 0.15 8.5430E-03 0.14
Rigid Bodies ......... 3.9456E-03 0.07 3.8590E-03 0.06
Time step size ....... 3.5202E-03 0.06 3.5140E-03 0.06
Rigid wall ........... 2.9770E-01 5.44 2.9668E-01 4.74
Group force file ..... 3.5784E-03 0.07 4.6710E-03 0.07
Others ............... 8.8898E-03 0.16 8.8780E-03 0.14
Misc. 1 .............. 2.5194E-01 4.60 2.5594E-01 4.09
Scale Masses ....... 2.3789E-03 0.04 2.4140E-03 0.04
Force Constraints .. 2.4560E-03 0.04 2.4670E-03 0.04
Force to Accel ..... 3.9114E-02 0.71 3.8768E-02 0.62
Update RB nodes .... 2.6085E-03 0.05 2.6180E-03 0.04
Misc. 2 .............. 6.7512E-02 1.23 6.6386E-02 1.06
Misc. 3 .............. 1.0380E-02 0.19 1.0283E-02 0.16
Misc. 4 .............. 2.2954E-02 0.42 2.3036E-02 0.37
Timestep Init ...... 1.3443E-02 0.25 1.3206E-02 0.21
Apply Loads ........ 4.6008E-03 0.08 4.7110E-03 0.08
----------------------------------------------------------------
T o t a l s 5.4758E+00 100.00 6.2532E+00 100.00
Problem time = 3.0004E-05
Problem cycle = 3225
Total CPU time = 5 seconds ( 0 hours 0 minutes 5 seconds)
CPU time per zone cycle = 348.068 nanoseconds
Clock time per zone cycle= 348.265 nanoseconds
Number of CPU's 1
NLQ used/max 112/ 112
Start time 11/04/2024 14:50:51
End time 11/04/2024 14:50:56
Elapsed time 5 seconds for 3225 cycles using 1 SMP thread
( 0 hour 0 minute 5 seconds)
N o r m a l t e r m i n a t i o n 11/04/24 14:50:56
Printed output to console
Get image and display#
def get_image_path(image_name):
return os.path.join(result_image_dir_server, image_name)
def display_image(path):
print(f"Printing {path} using matplotlib")
image1 = mpimg.imread(path)
plt.figure(figsize=(15, 15))
plt.axis("off")
plt.imshow(image1)
plt.show()
image_name = "totaldeformation.png"
image_path_server = get_image_path(image_name)
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
image_path_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
display_image(image_local_path)
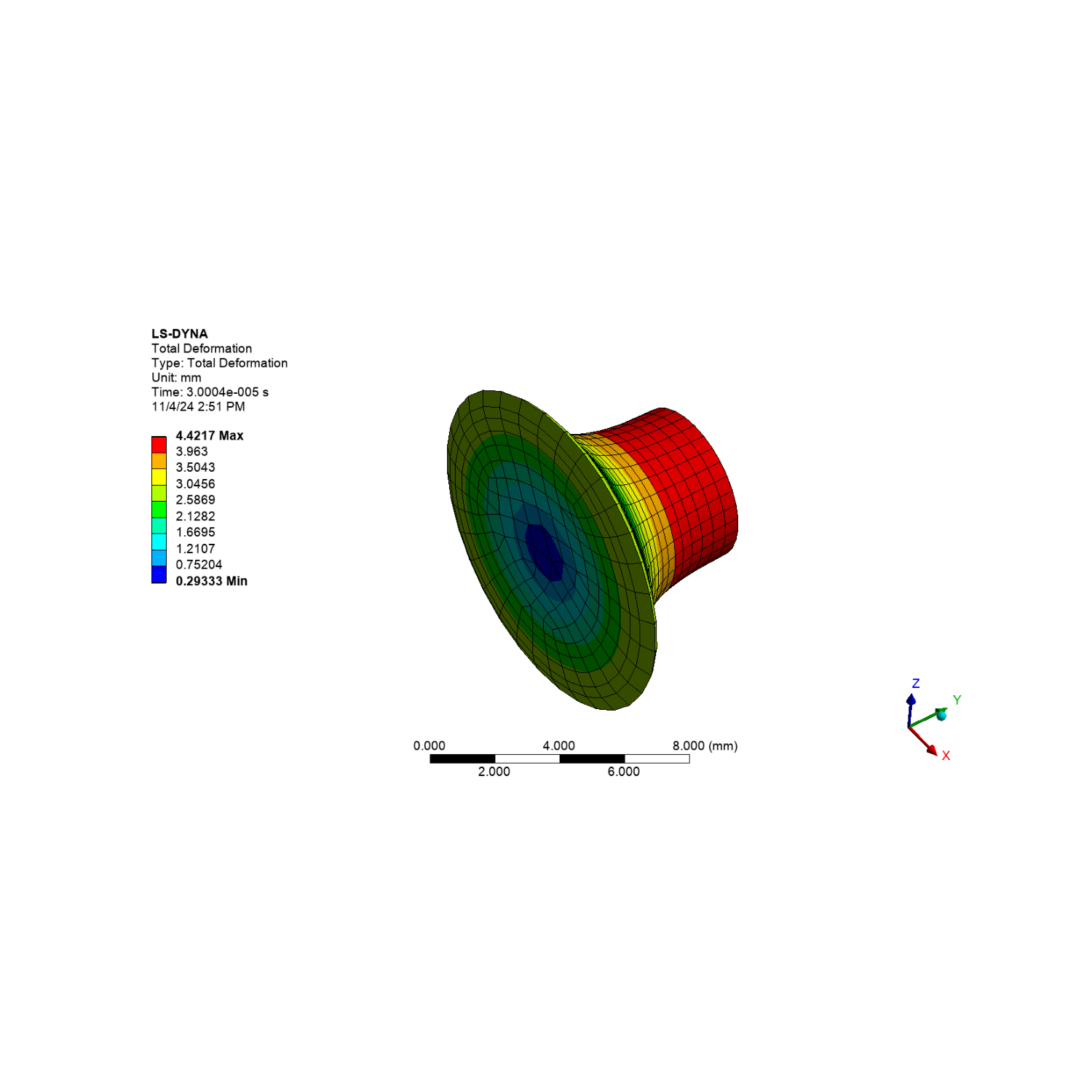
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/totaldeformation.png to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/totaldeformation.png: 0%| | 0.00/102k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/totaldeformation.png to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/totaldeformation.png: 100%|██████████| 102k/102k [00:00<00:00, 556MB/s]
Local image path : /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/totaldeformation.png
Printing /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/totaldeformation.png using matplotlib
Download gif and display#
animation_name = "taylor_bar.gif"
animation_server = get_image_path(animation_name)
def update(frame):
gif.seek(frame)
img.set_array(gif.convert("RGBA"))
return [img]
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
animation_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
gif = Image.open(image_local_path)
fig, ax = plt.subplots(figsize=(16, 9))
ax.axis("off")
img = ax.imshow(gif.convert("RGBA"))
ani = FuncAnimation(
fig, update, frames=range(gif.n_frames), interval=100, repeat=True, blit=True
)
plt.show()
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/taylor_bar.gif to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/taylor_bar.gif: 0%| | 0.00/10.1M [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech5176/Project_Mech_Files/ExplicitDynamics/taylor_bar.gif to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/taylor_bar.gif: 100%|██████████| 10.1M/10.1M [00:00<00:00, 236MB/s]
Local image path : /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/taylor_bar.gif
Close mechanical#
Close the mechanical instance.
print("Closing mechanical...")
mechanical.exit()
print("Mechanical closed!")
Closing mechanical...
Mechanical closed!
Total running time of the script: (0 minutes 20.773 seconds)