Note
Go to the end to download the full example code.
Bolt Pretension#
This example demonstrates how to insert a Static Structural analysis into a new Mechanical session and execute a sequence of Python scripting commands that define and solve a bolt-pretension analysis. Scripts then evaluate the following results: deformation, equivalent stresses, contact, and bolt
Import necessary libraries#
import os
from ansys.mechanical.core import launch_mechanical
from ansys.mechanical.core.examples import download_file
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
Launch mechanical#
Launch a new Mechanical session in batch, setting the cleanup_on_exit
argument to False
. To close this Mechanical session when finished,
this example must call the mechanical.exit()
method.
mechanical = launch_mechanical(batch=True, cleanup_on_exit=False)
print(mechanical)
Ansys Mechanical [Ansys Mechanical Enterprise]
Product Version:251
Software build date: 11/27/2024 09:34:44
Initialize variable for workflow#
Set the part_file_path
variable on the server for later use.
Make this variable compatible for Windows, Linux, and Docker containers.
project_directory = mechanical.project_directory
print(f"project directory = {project_directory}")
project directory = /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/
Download required geometry file#
Download the required file. Print the file path for the geometry file.
geometry_path = download_file(
"example_06_bolt_pret_geom.agdb", "pymechanical", "00_basic"
)
print(f"Downloaded the geometry file to: {geometry_path}")
Downloaded the geometry file to: /home/runner/.local/share/ansys_mechanical_core/examples/example_06_bolt_pret_geom.agdb
Upload the file to the project directory
mechanical.upload(file_name=geometry_path, file_location_destination=project_directory)
Uploading example_06_bolt_pret_geom.agdb to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/.: 0%| | 0.00/2.52M [00:00<?, ?B/s]
Uploading example_06_bolt_pret_geom.agdb to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/.: 100%|██████████| 2.52M/2.52M [00:00<00:00, 587MB/s]
'example_06_bolt_pret_geom.agdb'
Build the path relative to project directory and verify
base_name = os.path.basename(geometry_path)
combined_path = os.path.join(project_directory, base_name)
part_file_path = combined_path.replace("\\", "\\\\")
mechanical.run_python_script(f"part_file_path='{part_file_path}'")
result = mechanical.run_python_script("part_file_path")
print(f"Geometry file on server: {result}")
Geometry file on server: /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/example_06_bolt_pret_geom.agdb
Download required material file#
Download the required file. Print the file path for the material file
mat_cop_path = download_file("example_06_Mat_Copper.xml", "pymechanical", "00_basic")
print(f"Downloaded the material file to: {mat_cop_path}")
mat_st_path = download_file("example_06_Mat_Steel.xml", "pymechanical", "00_basic")
print(f"Downloaded the material file to: {mat_st_path}")
Downloaded the material file to: /home/runner/.local/share/ansys_mechanical_core/examples/example_06_Mat_Copper.xml
Downloaded the material file to: /home/runner/.local/share/ansys_mechanical_core/examples/example_06_Mat_Steel.xml
Upload the file to the project directory
mechanical.upload(file_name=mat_cop_path, file_location_destination=project_directory)
mechanical.upload(file_name=mat_st_path, file_location_destination=project_directory)
Uploading example_06_Mat_Copper.xml to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/.: 0%| | 0.00/5.00k [00:00<?, ?B/s]
Uploading example_06_Mat_Copper.xml to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/.: 100%|██████████| 5.00k/5.00k [00:00<00:00, 20.9MB/s]
Uploading example_06_Mat_Steel.xml to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/.: 0%| | 0.00/5.00k [00:00<?, ?B/s]
Uploading example_06_Mat_Steel.xml to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/.: 100%|██████████| 5.00k/5.00k [00:00<00:00, 27.3MB/s]
'example_06_Mat_Steel.xml'
Build the path relative to project directory and verify
base_name = os.path.basename(mat_cop_path)
combined_path = os.path.join(project_directory, base_name)
mat_Copper_file_path = combined_path.replace("\\", "\\\\")
mechanical.run_python_script(f"mat_Copper_file_path='{mat_Copper_file_path}'")
base_name = os.path.basename(mat_st_path)
combined_path = os.path.join(project_directory, base_name)
mat_Steel_file_path = combined_path.replace("\\", "\\\\")
mechanical.run_python_script(f"mat_Steel_file_path='{mat_Steel_file_path}'")
result = mechanical.run_python_script("mat_Copper_file_path")
print(f"mat_Copper_file_path on server: {result}")
result = mechanical.run_python_script("mat_Steel_file_path")
print(f"mat_Steel_file_path on server: {result}")
mat_Copper_file_path on server: /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/example_06_Mat_Copper.xml
mat_Steel_file_path on server: /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/example_06_Mat_Steel.xml
Run the script#
Run the Mechanical script to attach the geometry and set up and solve the analysis
output = mechanical.run_python_script(
"""
import json
import os
# Read geometry and material information.
geometry_import_group = Model.GeometryImportGroup
geometry_import = geometry_import_group.AddGeometryImport()
geometry_import_format = Ansys.Mechanical.DataModel.Enums.GeometryImportPreference.\
Format.Automatic
geometry_import_preferences = Ansys.ACT.Mechanical.Utilities.GeometryImportPreferences()
geometry_import_preferences.ProcessNamedSelections = True
geometry_import_preferences.ProcessCoordinateSystems = True
geometry_import.Import(part_file_path,geometry_import_format,geometry_import_preferences)
# Import materials.
MAT = ExtAPI.DataModel.Project.Model.Materials
MAT.Import(mat_Copper_file_path)
MAT.Import(mat_Steel_file_path)
Model.AddStaticStructuralAnalysis()
STAT_STRUC = Model.Analyses[0]
STAT_STRUC_SOLN = STAT_STRUC.Solution
STAT_STRUC_ANA_SETTING = STAT_STRUC.Children[0]
# Set up the unit system.
ExtAPI.Application.ActiveUnitSystem = MechanicalUnitSystem.StandardNMM
# Store all main tree nodes as variables.
MODEL = ExtAPI.DataModel.Project.Model
GEOM = ExtAPI.DataModel.Project.Model.Geometry
CONN_GRP = ExtAPI.DataModel.Project.Model.Connections
CS_GRP = ExtAPI.DataModel.Project.Model.CoordinateSystems
MSH = ExtAPI.DataModel.Project.Model.Mesh
NS_GRP = ExtAPI.DataModel.Project.Model.NamedSelections
# Store name selection.
block3_block2_cont_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block3_block2_cont'][0]
block3_block2_targ_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block3_block2_targ'][0]
shank_block3_targ_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_block3_targ'][0]
shank_block3_cont_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_block3_cont'][0]
block1_washer_cont_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block1_washer_cont'][0]
block1_washer_targ_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block1_washer_targ'][0]
washer_bolt_cont_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'washer_bolt_cont'][0]
washer_bolt_targ_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'washer_bolt_targ'][0]
shank_bolt_targ_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_bolt_targ'][0]
shank_bolt_cont_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_bolt_cont'][0]
block2_block1_cont_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block2_block1_cont'][0]
block2_block1_targ_NS = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block2_block1_targ'][0]
all_bodies = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'all_bodies'][0]
bodies_5 = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'bodies_5'][0]
shank = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank'][0]
shank_face = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_face'][0]
shank_face2 = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_face2'][0]
bottom_surface = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'bottom_surface'][0]
block2_surface = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'block2_surface'][0]
shank_surface = [x for x in ExtAPI.DataModel.Tree.AllObjects
if x.Name == 'shank_surface'][0]
# Assign material to bodies.
SURFACE1=GEOM.Children[0].Children[0]
SURFACE1.Material="Steel"
SURFACE2=GEOM.Children[1].Children[0]
SURFACE2.Material="Copper"
SURFACE3=GEOM.Children[2].Children[0]
SURFACE3.Material="Copper"
SURFACE4=GEOM.Children[3].Children[0]
SURFACE4.Material="Steel"
SURFACE5=GEOM.Children[4].Children[0]
SURFACE5.Material="Steel"
SURFACE6=GEOM.Children[5].Children[0]
SURFACE6.Material="Steel"
# Define coordinate system.
coordinate_systems_17 = Model.CoordinateSystems
coordinate_system_93 = coordinate_systems_17.AddCoordinateSystem()
coordinate_system_93.OriginDefineBy = CoordinateSystemAlignmentType.Fixed
coordinate_system_93.OriginX = Quantity(-195, "mm")
coordinate_system_93.OriginY = Quantity(100, "mm")
coordinate_system_93.OriginZ = Quantity(50, "mm")
coordinate_system_93.PrimaryAxis = CoordinateSystemAxisType.PositiveZAxis
# Change contact settings and add a command snippet to use the Archard Wear Model.
connections = ExtAPI.DataModel.Project.Model.Connections
# Delete existing contacts.
for connection in connections.Children:
if connection.DataModelObjectCategory==DataModelObjectCategory.ConnectionGroup:
connection.Delete()
CONT_REG1 = CONN_GRP.AddContactRegion()
CONT_REG1.SourceLocation = NS_GRP.Children[0]
CONT_REG1.TargetLocation = NS_GRP.Children[1]
CONT_REG1.ContactType=ContactType.Frictional
CONT_REG1.FrictionCoefficient = 0.2
CONT_REG1.SmallSliding = ContactSmallSlidingType.Off
CONT_REG1.UpdateStiffness = UpdateContactStiffness.Never
CMD1=CONT_REG1.AddCommandSnippet()
# Add missing contact keyopt and Archard Wear Model in workbench using a command snippet.
AWM = '''keyopt,cid,9,5
rmodif,cid,10,0.00
rmodif,cid,23,0.001'''
CMD1.AppendText(AWM)
CONTS = CONN_GRP.Children[0]
CONT_REG2 = CONTS.AddContactRegion()
CONT_REG2.SourceLocation = NS_GRP.Children[3]
CONT_REG2.TargetLocation = NS_GRP.Children[2]
CONT_REG2.ContactType=ContactType.Bonded
CONT_REG2.ContactFormulation = ContactFormulation.MPC
CONT_REG3 = CONTS.AddContactRegion()
CONT_REG3.SourceLocation = NS_GRP.Children[4]
CONT_REG3.TargetLocation = NS_GRP.Children[5]
CONT_REG3.ContactType=ContactType.Frictional
CONT_REG3.FrictionCoefficient = 0.2
CONT_REG3.SmallSliding = ContactSmallSlidingType.Off
CONT_REG3.UpdateStiffness = UpdateContactStiffness.Never
CMD3=CONT_REG3.AddCommandSnippet()
# Add missing contact keyopt and Archard Wear Model in workbench using a command snippet.
AWM3 = '''keyopt,cid,9,5
rmodif,cid,10,0.00
rmodif,cid,23,0.001'''
CMD3.AppendText(AWM3)
CONT_REG4 = CONTS.AddContactRegion()
CONT_REG4.SourceLocation = NS_GRP.Children[6]
CONT_REG4.TargetLocation = NS_GRP.Children[7]
CONT_REG4.ContactType=ContactType.Bonded
CONT_REG4.ContactFormulation = ContactFormulation.MPC
CONT_REG5 = CONTS.AddContactRegion()
CONT_REG5.SourceLocation = NS_GRP.Children[9]
CONT_REG5.TargetLocation = NS_GRP.Children[8]
CONT_REG5.ContactType=ContactType.Bonded
CONT_REG5.ContactFormulation = ContactFormulation.MPC
CONT_REG6 = CONTS.AddContactRegion()
CONT_REG6.SourceLocation = NS_GRP.Children[10]
CONT_REG6.TargetLocation = NS_GRP.Children[11]
CONT_REG6.ContactType=ContactType.Frictional
CONT_REG6.FrictionCoefficient = 0.2
CONT_REG6.SmallSliding = ContactSmallSlidingType.Off
CONT_REG6.UpdateStiffness = UpdateContactStiffness.Never
CMD6=CONT_REG6.AddCommandSnippet()
# Add missing contact keyopt and Archard Wear Model in workbench using a command snippet.
AWM6 = '''keyopt,cid,9,5
rmodif,cid,10,0.00
rmodif,cid,23,0.001'''
CMD6.AppendText(AWM6)
# Add contact tool.
#CONT_TOOL = CONN_GRP.AddContactTool()
#CONT_TOOL.AddPenetration()
#CONT_TOOL.AddStatus()
#CONT_TOOL.GenerateInitialContactResults()
# Generate mesh.
Hex_Method = MSH.AddAutomaticMethod()
Hex_Method.Location = all_bodies
Hex_Method.Method = MethodType.HexDominant
BODY_SIZING1=MSH.AddSizing()
BODY_SIZING1.Location=bodies_5
BODY_SIZING1.ElementSize = Quantity(15, "mm")
BODY_SIZING2=MSH.AddSizing()
BODY_SIZING2.Location=shank
BODY_SIZING2.ElementSize = Quantity(7, "mm")
Face_Meshing = MSH.AddFaceMeshing()
Face_Meshing.Location = shank_face
Face_Meshing.MappedMesh = False
Sweep_Method = MSH.AddAutomaticMethod()
Sweep_Method.Location = shank
Sweep_Method.Method = MethodType.Sweep
Sweep_Method.SourceTargetSelection = 2
Sweep_Method.SourceLocation = shank_face
Sweep_Method.TargetLocation = shank_face2
MSH.GenerateMesh()
# Set up analysis settings.
STAT_STRUC_ANA_SETTING.NumberOfSteps = 4
step_index_list = [1]
with Transaction():
for step_index in step_index_list:
STAT_STRUC_ANA_SETTING.SetAutomaticTimeStepping(step_index, AutomaticTimeStepping.Off)
STAT_STRUC_ANA_SETTING.Activate()
step_index_list = [1]
with Transaction():
for step_index in step_index_list:
STAT_STRUC_ANA_SETTING.SetNumberOfSubSteps(step_index, 2)
STAT_STRUC_ANA_SETTING.Activate()
STAT_STRUC_ANA_SETTING.SolverType = SolverType.Direct
STAT_STRUC_ANA_SETTING.SolverPivotChecking = SolverPivotChecking.Off
# Insert loading and BC.
FIX_SUP=STAT_STRUC.AddFixedSupport()
FIX_SUP.Location=block2_surface
Tabular_Force = STAT_STRUC.AddForce()
Tabular_Force.Location = bottom_surface
Tabular_Force.DefineBy = LoadDefineBy.Components
Tabular_Force.XComponent.Inputs[0].DiscreteValues = [Quantity('0[s]'),Quantity('1[s]'), \
Quantity('2[s]'),Quantity('3[s]'),Quantity('4[s]')]
Tabular_Force.XComponent.Output.DiscreteValues = [Quantity('0[N]'),Quantity('0[N]'), \
Quantity('5.e+005[N]'),Quantity('0[N]'),Quantity('-5.e+005[N]')]
Bolt_Pretension = STAT_STRUC.AddBoltPretension()
Bolt_Pretension.Location = shank_surface
Bolt_Pretension.Preload.Inputs[0].DiscreteValues = [Quantity('1[s]'),Quantity('2[s]'), \
Quantity('3[s]'),Quantity('4[s]')]
Bolt_Pretension.Preload.Output.DiscreteValues = [Quantity('6.1363e+005[N]'), \
Quantity('0 [N]'),Quantity('0 [N]'),Quantity('0[N]')]
Bolt_Pretension.SetDefineBy(2,BoltLoadDefineBy.Lock)
Bolt_Pretension.SetDefineBy(3,BoltLoadDefineBy.Lock)
Bolt_Pretension.SetDefineBy(4,BoltLoadDefineBy.Lock)
# Insert results.
Post_Contact_Tool = STAT_STRUC_SOLN.AddContactTool()
Post_Contact_Tool.ScopingMethod = GeometryDefineByType.Worksheet
Bolt_Tool = STAT_STRUC_SOLN.AddBoltTool()
Bolt_Working_Load = Bolt_Tool.AddWorkingLoad()
Total_Deformation = STAT_STRUC_SOLN.AddTotalDeformation()
Equivalent_stress_1 = STAT_STRUC_SOLN.AddEquivalentStress()
Equivalent_stress_2 = STAT_STRUC_SOLN.AddEquivalentStress()
Equivalent_stress_2.Location = shank
Force_Reaction_1 = STAT_STRUC_SOLN.AddForceReaction()
Force_Reaction_1.BoundaryConditionSelection = FIX_SUP
Moment_Reaction_2 = STAT_STRUC_SOLN.AddMomentReaction()
Moment_Reaction_2.BoundaryConditionSelection = FIX_SUP
# Set number of processors to 6 using DANSYS. (Optional)
# Num_Cores = STAT_STRUC.SolveConfiguration.SolveProcessSettings.MaxNumberOfCores
# STAT_STRUC.SolveConfiguration.SolveProcessSettings.MaxNumberOfCores = 6
# Solve and validate the results.
STAT_STRUC_SOLN.Solve(True)
STAT_STRUC_SS=STAT_STRUC_SOLN.Status
# Set the isometric view and zoom to fit.
cam = Graphics.Camera
cam.SetSpecificViewOrientation(ViewOrientationType.Iso)
cam.SetFit()
mechdir = STAT_STRUC.Children[0].SolverFilesDirectory
export_path = os.path.join(mechdir, "contact_status.png")
Post_Contact_Tool.Children[0].Activate()
Graphics.ExportImage(export_path, GraphicsImageExportFormat.PNG)
my_results_details = {
"Total_Deformation": str(Total_Deformation.Maximum),
"Equivalent_Stress1": str(Equivalent_stress_1.Maximum),
"Equivalent_Stress2": str(Equivalent_stress_2.Maximum),
}
json.dumps(my_results_details)
"""
)
print(output)
{"Equivalent_Stress1": "1947.4823628435474 [MPa]", "Total_Deformation": "0.38461775757867667 [mm]", "Equivalent_Stress2": "1947.4823628435474 [MPa]"}
Initialize the variable needed for the image directory#
Set the image_dir
variable for later use.
Make the variable compatible for Windows, Linux, and Docker containers.
# image_directory_modified = project_directory.replace("\\", "\\\\")
mechanical.run_python_script(f"image_dir=ExtAPI.DataModel.AnalysisList[0].WorkingDir")
# Verify the path for image directory.
result_image_dir_server = mechanical.run_python_script(f"image_dir")
print(f"Images are stored on the server at: {result_image_dir_server}")
Images are stored on the server at: /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/
Download the image and plot#
Download one image file from the server to the current working directory and plot using matplotlib.
def get_image_path(image_name):
return os.path.join(result_image_dir_server, image_name)
def display_image(path):
print(f"Printing {path} using matplotlib")
image1 = mpimg.imread(path)
plt.figure(figsize=(15, 15))
plt.axis("off")
plt.imshow(image1)
plt.show()
image_name = "contact_status.png"
image_path_server = get_image_path(image_name)
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
image_path_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
display_image(image_local_path)
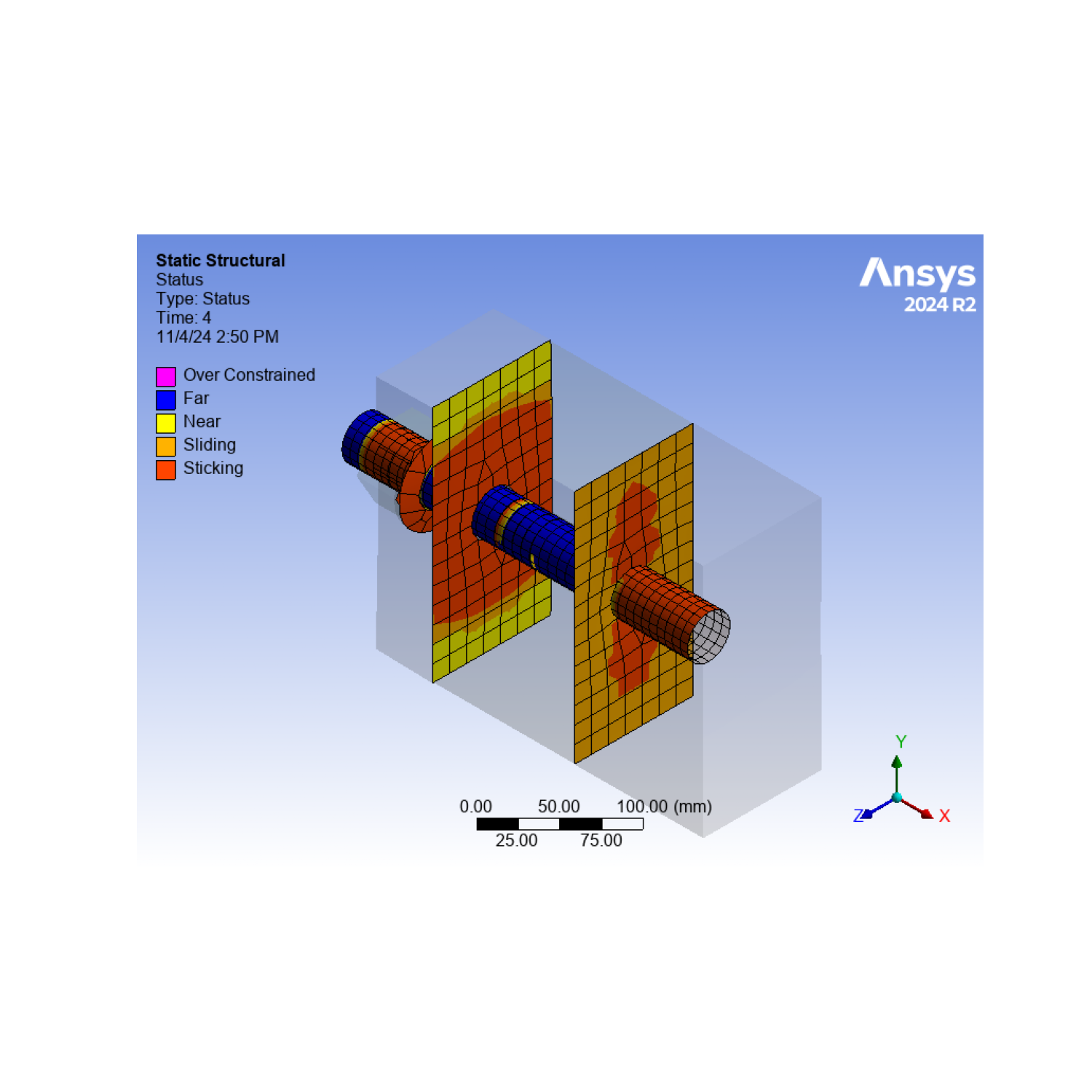
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/contact_status.png to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/contact_status.png: 0%| | 0.00/74.5k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/contact_status.png to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/contact_status.png: 100%|██████████| 74.5k/74.5k [00:00<00:00, 399MB/s]
Local image path : /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/contact_status.png
Printing /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/contact_status.png using matplotlib
Download output file from solve and print contents#
Download the solve.out
file from the server to the current working
directory and print the contents. Remove the solve.out
file.
def get_solve_out_path(mechanical):
"""Get the solve out path and return."""
solve_out_path = ""
for file_path in mechanical.list_files():
if file_path.find("solve.out") != -1:
solve_out_path = file_path
break
return solve_out_path
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
solve_out_path = get_solve_out_path(mechanical)
if solve_out_path != "":
current_working_directory = os.getcwd()
mechanical.download(solve_out_path, target_dir=current_working_directory)
solve_out_local_path = os.path.join(current_working_directory, "solve.out")
write_file_contents_to_console(solve_out_local_path)
os.remove(solve_out_local_path)
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/solve.out to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/solve.out: 0%| | 0.00/78.8k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/solve.out to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/basic/solve.out: 100%|██████████| 78.8k/78.8k [00:00<00:00, 388MB/s]
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2025 R1 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2025 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
2025 R1
Point Releases and Patches installed:
Ansys, Inc. License Manager 2025 R1
LS-DYNA 2025 R1
Core WB Files 2025 R1
Mechanical Products 2025 R1
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
DISTRIBUTED MEMORY PARALLEL REQUESTED
4 PARALLEL PROCESSES REQUESTED WITH SINGLE THREAD PER PROCESS
TOTAL OF 4 CORES REQUESTED
INPUT FILE NAME = /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/dummy.dat
OUTPUT FILE NAME = /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2025 R1 BUILD= 25.1 UP20241202 VERSION=LINUX x64
CURRENT JOBNAME=file0 06:45:36 APR 14, 2025 CP= 0.243
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
*** NOTE *** CP = 0.348 TIME= 06:45:36
The /CONFIG,NOELDB command is not valid in a distributed memory
parallel solution. Command is ignored.
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 6.76000000
TITLE=
--Static Structural
ACT Extensions:
LSDYNA, 2025.1
5f463412-bd3e-484b-87e7-cbc0a665e474, wbex
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/UserFiles/
--- Data in consistent NMM units. See Solving Units in the help system for more
MPA UNITS SPECIFIED FOR INTERNAL
LENGTH = MILLIMETERS (mm)
MASS = TONNE (Mg)
TIME = SECONDS (sec)
TEMPERATURE = CELSIUS (C)
TOFFSET = 273.0
FORCE = NEWTON (N)
HEAT = MILLIJOULES (mJ)
INPUT UNITS ARE ALSO SET TO MPA
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2025 R1 25.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 06:45:36 APR 14, 2025 CP= 0.352
--Static Structural
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Nodes for all Remote Points ***********
*********** Elements for Body 1 'Solid' ***********
*********** Elements for Body 3 'Solid' ***********
*********** Elements for Body 5 'Solid' ***********
*********** Elements for Body 7 'Solid' ***********
*********** Elements for Body 9 'Solid' ***********
*********** Elements for Body 11 'Solid' ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Create Contact "Contact Region" ***********
Real Constant Set For Above Contact Is 13 & 12
*********** Create Contact "Contact Region 2" ***********
Real Constant Set For Above Contact Is 15 & 14
*********** Create Contact "Contact Region 3" ***********
Real Constant Set For Above Contact Is 17 & 16
*********** Create Contact "Contact Region 4" ***********
Real Constant Set For Above Contact Is 19 & 18
*********** Create Contact "Contact Region 5" ***********
Real Constant Set For Above Contact Is 21 & 20
*********** Create Contact "Contact Region 6" ***********
Real Constant Set For Above Contact Is 23 & 22
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Element Component ***********
*********** Send Named Selection as Element Component ***********
*********** Send Named Selection as Element Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Fixed Supports ***********
*********** Define Force Using Surface Effect Elements ***********
*********** Create Bolt Pretension "Bolt Pretension" ***********
CREATE COMPONENT CMEBOLT FROM PRETENSION ELEMENTS
CREATE COMPONENT CMNBOLT FROM PRETENSION NODES
***** ROUTINE COMPLETED ***** CP = 1.028
--- Number of total nodes = 20313
--- Number of contact elements = 4628
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 4403
--- Number of condensed parts = 0
--- Number of total elements = 9161
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 6.76027778
****************************************************************************
************************* SOLUTION ********************************
****************************************************************************
***** MAPDL SOLUTION ROUTINE *****
PERFORM A STATIC ANALYSIS
THIS WILL BE A NEW ANALYSIS
EQUATION PIVOT CHECKING LOGIC WILL BE DISABLED
PRINT EQUATION PIVOT INFO ONCE PER LOADSTEP
PARAMETER _THICKRATIO = 0.6670000000
USE SPARSE MATRIX DIRECT SOLVER
CONTACT INFORMATION PRINTOUT LEVEL 1
DO NOT COMBINE ELEMENT MATRIX FILES (.emat) AFTER DISTRIBUTED PARALLEL SOLUTION
DO NOT COMBINE ELEMENT SAVE DATA FILES (.esav) AFTER DISTRIBUTED PARALLEL SOLUTION
NLDIAG: Nonlinear diagnostics CONT option is set to ON.
Writing frequency : each ITERATION.
DEFINE RESTART CONTROL FOR LOADSTEP LAST
AT FREQUENCY OF LAST AND NUMBER FOR OVERWRITE IS -1
DELETE RESTART FILES OF ENDSTEP
****************************************************
******************* SOLVE FOR LS 1 OF 4 ****************
SELECT FOR ITEM=TYPE COMPONENT=
IN RANGE 24 TO 24 STEP 1
98 ELEMENTS (OF 9161 DEFINED) SELECTED BY ESEL COMMAND.
SELECT ALL NODES HAVING ANY ELEMENT IN ELEMENT SET.
337 NODES (OF 20313 DEFINED) SELECTED FROM
98 SELECTED ELEMENTS BY NSLE COMMAND.
SPECIFIED SURFACE LOAD PRES FOR ALL SELECTED ELEMENTS LKEY = 1 KVAL = 1
SET ACCORDING TO TABLE PARAMETER = _LOADVARI153X
SPECIFIED SURFACE LOAD PRES FOR ALL SELECTED ELEMENTS LKEY = 2 KVAL = 1
VALUES = 0.0000 0.0000 0.0000 0.0000
SPECIFIED SURFACE LOAD PRES FOR ALL SELECTED ELEMENTS LKEY = 3 KVAL = 1
VALUES = 0.0000 0.0000 0.0000 0.0000
ALL SELECT FOR ITEM=NODE COMPONENT=
IN RANGE 1 TO 20313 STEP 1
20313 NODES (OF 20313 DEFINED) SELECTED BY NSEL COMMAND.
ALL SELECT FOR ITEM=ELEM COMPONENT=
IN RANGE 1 TO 11818 STEP 1
9161 ELEMENTS (OF 9161 DEFINED) SELECTED BY ESEL COMMAND.
PRINTOUT RESUMED BY /GOP
SPECIFIED NODAL LOAD FX FOR SELECTED NODES 20183 TO 20183 BY 1
REAL= 613630.000 IMAG= 0.00000000
DO NOT USE AUTOMATIC TIME STEPPING THIS LOAD STEP
USE 2 SUBSTEPS INITIALLY THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
FOR AUTOMATIC TIME STEPPING:
USE 2 SUBSTEPS AS A MAXIMUM
USE 2 SUBSTEPS AS A MINIMUM
TIME= 1.0000
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPPL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
*** NOTE *** CP = 1.135 TIME= 06:45:37
The automatic domain decomposition logic has selected the MESH domain
decomposition method with 4 processes per solution.
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 1.171 TIME= 06:45:37
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 1.219 TIME= 06:45:37
The model data was checked and warning messages were found.
Please review output or errors file (
/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/fil
le0.err ) for these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID186. KEYOPT(2)=0 IS SUGGESTED AND HAS BEEN RESET.
KEYOPT(1-12)= 0 0 0 0 0 0 0 0 0 0 0 0
ELEMENT TYPE 3 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 4 IS SOLID186. KEYOPT(2)=0 IS SUGGESTED AND HAS BEEN RESET.
KEYOPT(1-12)= 0 0 0 0 0 0 0 0 0 0 0 0
ELEMENT TYPE 5 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 6 IS SOLID186. KEYOPT(2)=0 IS SUGGESTED AND HAS BEEN RESET.
KEYOPT(1-12)= 0 0 0 0 0 0 0 0 0 0 0 0
ELEMENT TYPE 7 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 8 IS SOLID186. KEYOPT(2)=0 IS SUGGESTED AND HAS BEEN RESET.
KEYOPT(1-12)= 0 0 0 0 0 0 0 0 0 0 0 0
ELEMENT TYPE 9 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 10 IS SOLID186. KEYOPT(2)=0 IS SUGGESTED AND HAS BEEN RESET.
KEYOPT(1-12)= 0 0 0 0 0 0 0 0 0 0 0 0
ELEMENT TYPE 11 IS SOLID186. KEYOPT(2)=0 IS SUGGESTED AND HAS BEEN RESET.
KEYOPT(1-12)= 0 0 0 0 0 0 0 0 0 0 0 0
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2025 R1 25.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 06:45:37 APR 14, 2025 CP= 1.229
--Static Structural
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .STATIC (STEADY-STATE)
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
EQUATION SOLVER OPTION. . . . . . . . . . . . .SPARSE
NEWTON-RAPHSON OPTION . . . . . . . . . . . . .PROGRAM CHOSEN
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .SYMMETRIC
*** WARNING *** CP = 1.270 TIME= 06:45:37
Material number 24 (used by element 11591) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 1.283 TIME= 06:45:37
The step data was checked and warning messages were found.
Please review output or errors file (
/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/fil
le0.err ) for these warning messages.
*** NOTE *** CP = 1.283 TIME= 06:45:37
This nonlinear analysis defaults to using the full Newton-Raphson
solution procedure. This can be modified using the NROPT command.
*WARNING*: Some MPC/Lagrange based elements (e.g.7541) in real constant
set 15 overlap with other MPC/Lagrange based elements (e.g.9535) in
real constant set 21 which can cause overconstraint.
*** NOTE *** CP = 2.281 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 12 and contact element type 12 has been set up. The
companion pair has real constant set ID 13. Both pairs should have
the same behavior.
*WARNING*: The contact pairs have similar mesh patterns which can cause
overconstraint. MAPDL will deactivate the current pair and keep its
companion pair.
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 1.0000
The resulting initial contact stiffness 0.18456E+06
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 1.3817
Max. initial friction coefficient MU 0.20000
Default tangent stiffness factor FKT 1.0000
*** WARNING *** CP = 2.281 TIME= 06:45:37
It is highly recommended to set KEYOPT(10)=0 (update contact stiffness
at each iteration) in order to achieve better convergence.
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 14.016
Average contact pair depth 13.817
Average target surface length 13.943
Default pinball region factor PINB 1.0000
The resulting pinball region 13.817
Contact offset is included. Initial geometric penetration is excluded.
*** NOTE *** CP = 2.281 TIME= 06:45:37
Max. Initial penetration 0 was detected between contact element 7161
and target element 7367.
****************************************
*** NOTE *** CP = 2.281 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 13 and contact element type 12 has been set up. The
companion pair has real constant set ID 12. Both pairs should have
the same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 1.0000
The resulting initial contact stiffness 0.18456E+06
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 1.4088
Max. initial friction coefficient MU 0.20000
Default tangent stiffness factor FKT 1.0000
*WARNING*: It is highly recommended to set KEYOPT(10)=0 (update contact
stiffness at each iteration) in order to achieve better convergence.
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 14.007
Average contact pair depth 14.088
Average target surface length 13.942
Default pinball region factor PINB 1.0000
The resulting pinball region 14.088
Contact offset is included. Initial geometric penetration is excluded.
*** NOTE *** CP = 2.282 TIME= 06:45:37
Max. Initial penetration 0 was detected between contact element 7261
and target element 7063.
****************************************
*** NOTE *** CP = 2.282 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 14 and contact element type 14 has been set up. The
companion pair has real constant set ID 15. Both pairs should have
the same behavior.
MAPDL will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 2.282 TIME= 06:45:37
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 13.531
Average contact pair depth 9.0953
Average target surface length 6.3865
Default pinball region factor PINB 0.25000
The resulting pinball region 2.2738
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 2.282 TIME= 06:45:37
Max. Initial penetration 0.684961413 was detected between contact
element 7530 and target element 8552.
You may move entire target surface by : x= 3.574949984E-16, y=
-0.262123383, z= 0.632821831,to reduce initial penetration.
****************************************
*** NOTE *** CP = 2.282 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 15 and contact element type 14 has been set up. The
companion pair has real constant set ID 14. Both pairs should have
the same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Distributed constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 2.282 TIME= 06:45:37
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
*WARNING*: Certain contact elements (for example 8368&10362) overlap
each other. Overconstraint may occur.
Average contact surface length 6.4480
Average contact pair depth 5.1166
Average target surface length 13.388
Default pinball region factor PINB 0.25000
The resulting pinball region 1.2792
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 2.282 TIME= 06:45:37
Max. Initial penetration 0.675803228 was detected between contact
element 7816 and target element 7491.
You may move entire target surface by : x= 3.618027317E-02, y=
-0.234296751, z= -0.632855452,to reduce initial penetration.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 2.282 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 16 and contact element type 16 has been set up. The
companion pair has real constant set ID 17. Both pairs should have
the same behavior.
MAPDL will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 1.0000
The resulting initial contact stiffness 0.21483E+06
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 1.2103
Max. initial friction coefficient MU 0.20000
Default tangent stiffness factor FKT 1.0000
*WARNING*: It is highly recommended to set KEYOPT(10)=0 (update contact
stiffness at each iteration) in order to achieve better convergence.
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 14.017
Average contact pair depth 12.103
Average target surface length 14.211
Default pinball region factor PINB 1.0000
The resulting pinball region 12.103
Contact offset is included. Initial geometric penetration is excluded.
*** NOTE *** CP = 2.282 TIME= 06:45:37
Max. Initial penetration 0 was detected between contact element 9297
and target element 9425.
****************************************
*** NOTE *** CP = 2.282 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 17 and contact element type 16 has been set up. The
companion pair has real constant set ID 16. Both pairs should have
the same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 1.0000
The resulting initial contact stiffness 0.21483E+06
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 0.44258
Max. initial friction coefficient MU 0.20000
Default tangent stiffness factor FKT 1.0000
*WARNING*: It is highly recommended to set KEYOPT(10)=0 (update contact
stiffness at each iteration) in order to achieve better convergence.
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 14.576
Average contact pair depth 4.4258
Average target surface length 13.943
Default pinball region factor PINB 1.0000
The resulting pinball region 4.4258
Contact offset is included. Initial geometric penetration is excluded.
*** NOTE *** CP = 2.282 TIME= 06:45:37
Max. Initial penetration 0 was detected between contact element 9397
and target element 9246.
****************************************
*** NOTE *** CP = 2.282 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 18 and contact element type 18 has been set up. The
companion pair has real constant set ID 19. Both pairs should have
the same behavior.
MAPDL will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 2.282 TIME= 06:45:37
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 14.393
Average contact pair depth 4.4329
Average target surface length 12.238
Default pinball region factor PINB 0.25000
The resulting pinball region 1.1082
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 2.282 TIME= 06:45:37
Max. Initial penetration 5.684341886E-14 was detected between contact
element 9445 and target element 9477.
****************************************
*** NOTE *** CP = 2.282 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 19 and contact element type 18 has been set up. The
companion pair has real constant set ID 18. Both pairs should have
the same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Distributed constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 2.283 TIME= 06:45:37
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
*WARNING*: Certain contact elements (for example 9471&9523) overlap
each other. Overconstraint may occur.
Average contact surface length 12.576
Average contact pair depth 12.199
Average target surface length 14.266
Default pinball region factor PINB 0.25000
The resulting pinball region 3.0498
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 2.283 TIME= 06:45:37
Max. Initial penetration 1.136868377E-13 was detected between contact
element 9458 and target element 9432.
****************************************
*** NOTE *** CP = 2.283 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 20 and contact element type 20 has been set up. The
companion pair has real constant set ID 21. Both pairs should have
the same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Distributed constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 2.283 TIME= 06:45:37
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
*WARNING*: Certain contact elements (for example 9526&9463) overlap
each other. Overconstraint may occur.
Average contact surface length 13.088
Average contact pair depth 6.7345
Average target surface length 6.3865
Default pinball region factor PINB 0.25000
The resulting pinball region 1.6836
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 2.283 TIME= 06:45:37
Max. Initial penetration 1.28495889 was detected between contact
element 9514 and target element 11104.
You may move entire target surface by : x= 1.224539025E-15, y=
-1.18655332, z= -0.493163843,to reduce initial penetration.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 2.283 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 21 and contact element type 20 has been set up. The
companion pair has real constant set ID 20. Both pairs should have
the same behavior.
MAPDL will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Distributed constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 2.283 TIME= 06:45:37
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 6.4480
Average contact pair depth 5.1166
Average target surface length 12.889
Default pinball region factor PINB 0.25000
The resulting pinball region 1.2792
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 2.283 TIME= 06:45:37
Max. Initial penetration 1.2132628 was detected between contact
element 10276 and target element 9490.
You may move entire target surface by : x= 2.447930752E-02, y=
1.1355368, z= 0.426571867,to reduce initial penetration.
*WARNING*: The detected Max. Penetration is close to the pair based
pinball. Please verify the pinball radius carefully.
*WARNING*: The geometric gap/penetration may be too large. Increase
pinball radius if it is a true geometric gap/penetration. Decrease
pinball if it is a false one.
****************************************
*** NOTE *** CP = 2.283 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 22 and contact element type 22 has been set up. The
companion pair has real constant set ID 23. Both pairs should have
the same behavior.
*WARNING*: The contact pairs have similar mesh patterns which can cause
overconstraint. MAPDL will deactivate the current pair and keep its
companion pair.
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 1.0000
The resulting initial contact stiffness 0.18193E+06
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 1.4291
Max. initial friction coefficient MU 0.20000
Default tangent stiffness factor FKT 1.0000
*WARNING*: It is highly recommended to set KEYOPT(10)=0 (update contact
stiffness at each iteration) in order to achieve better convergence.
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 14.016
Average contact pair depth 14.291
Average target surface length 13.943
Default pinball region factor PINB 1.0000
The resulting pinball region 14.291
Contact offset is included. Initial geometric penetration is excluded.
*** NOTE *** CP = 2.283 TIME= 06:45:37
Max. Initial penetration 0 was detected between contact element 11291
and target element 11491.
****************************************
*** NOTE *** CP = 2.283 TIME= 06:45:37
Symmetric Deformable- deformable contact pair identified by real
constant set 23 and contact element type 22 has been set up. The
companion pair has real constant set ID 22. Both pairs should have
the same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Contact algorithm: Augmented Lagrange method
Contact detection at: Gauss integration point
Contact stiffness factor FKN 1.0000
The resulting initial contact stiffness 0.18193E+06
Default penetration tolerance factor FTOLN 0.10000
The resulting penetration tolerance 1.2341
Max. initial friction coefficient MU 0.20000
Default tangent stiffness factor FKT 1.0000
*WARNING*: It is highly recommended to set KEYOPT(10)=0 (update contact
stiffness at each iteration) in order to achieve better convergence.
Use constant contact stiffness
Default Max. friction stress TAUMAX 0.10000E+21
Average contact surface length 14.007
Average contact pair depth 12.341
Average target surface length 13.942
Default pinball region factor PINB 1.0000
The resulting pinball region 12.341
Contact offset is included. Initial geometric penetration is excluded.
*** NOTE *** CP = 2.283 TIME= 06:45:37
Max. Initial penetration 0 was detected between contact element 11391
and target element 11191.
****************************************
*** WARNING *** CP = 2.283 TIME= 06:45:37
Overconstraint may occur for Lagrange multiplier or MPC based contact
algorithm.
The reasons for possible overconstraint are:
*** WARNING *** CP = 2.283 TIME= 06:45:37
Certain contact elements (for example 9526 & 9463) overlap with other.
****************************************
D I S T R I B U T E D D O M A I N D E C O M P O S E R
...Number of elements: 9161
...Number of nodes: 20313
...Decompose to 4 CPU domains
...Element load balance ratio = 1.668
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
TIME AT END OF THE LOAD STEP. . . . . . . . . . 1.0000
NUMBER OF SUBSTEPS. . . . . . . . . . . . . . . 2
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS. . . . 15
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . NO
TERMINATE ANALYSIS IF NOT CONVERGED . . . . . .YES (EXIT)
CONVERGENCE CONTROLS. . . . . . . . . . . . . .USE DEFAULTS
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
RSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
EPPL ALL
CONT ALL
SOLUTION MONITORING INFO IS WRITTEN TO FILE= file.mntr
>>>> PRETENSION ELEMENT STATUS <<<<
SECTION NAME PT.NODE --------STATUS-------------------- ----LOAD SOURCE----
25 20183 APPLIED PRELOAD FORCE= 0.61363E+06 F COMMAND
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS HAS BEEN MODIFIED
TO BE, NEQIT = 26, BY SOLUTION CONTROL LOGIC.
Range of element maximum matrix coefficients in global coordinates
Maximum = 29991749.6 at element 2586.
Minimum = 820595.982 at element 1132.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 80 SOLID187 0.005 0.000059
2 976 SOLID186 0.114 0.000117
3 79 SOLID187 0.005 0.000066
4 985 SOLID186 0.120 0.000122
5 10 SOLID187 0.001 0.000059
6 448 SOLID186 0.054 0.000121
7 10 SOLID187 0.001 0.000064
8 51 SOLID186 0.006 0.000126
9 4 SOLID187 0.000 0.000072
10 58 SOLID186 0.008 0.000138
11 1702 SOLID186 0.203 0.000120
12 200 CONTA174 0.041 0.000205
13 200 TARGE170 0.001 0.000004
14 868 CONTA174 0.029 0.000034
15 868 TARGE170 0.005 0.000006
16 115 CONTA174 0.007 0.000064
17 115 TARGE170 0.001 0.000006
18 30 CONTA174 0.006 0.000187
19 30 TARGE170 0.000 0.000005
20 852 CONTA174 0.027 0.000032
21 852 TARGE170 0.004 0.000005
22 200 CONTA174 0.041 0.000203
23 200 TARGE170 0.001 0.000005
24 98 SURF154 0.004 0.000037
25 130 PRETS179 0.000 0.000003
Time at end of element matrix formulation CP = 3.89866281.
ALL CURRENT MAPDL DATA WRITTEN TO FILE NAME= file.rdb
FOR POSSIBLE RESUME FROM THIS POINT
FORCE CONVERGENCE VALUE = 0.3068E+06 CRITERION= 1534.
DISTRIBUTED SPARSE MATRIX DIRECT SOLVER.
Number of equations = 55198, Maximum wavefront = 915
Memory allocated on only this MPI rank (rank 0)
-------------------------------------------------------------------
Equation solver memory allocated = 142.596 MB
Equation solver memory required for in-core mode = 136.807 MB
Equation solver memory required for out-of-core mode = 60.815 MB
Total (solver and non-solver) memory allocated = 862.701 MB
Total memory summed across all MPI ranks on this machines
-------------------------------------------------------------------
Equation solver memory allocated = 548.643 MB
Equation solver memory required for in-core mode = 526.391 MB
Equation solver memory required for out-of-core mode = 216.805 MB
Total (solver and non-solver) memory allocated = 2477.878 MB
*** NOTE *** CP = 4.272 TIME= 06:45:38
The Distributed Sparse Matrix Solver is currently running in the
in-core memory mode. This memory mode uses the most amount of memory
in order to avoid using the hard drive as much as possible, which most
often results in the fastest solution time. This mode is recommended
if enough physical memory is present to accommodate all of the solver
data.
curEqn= 14497 totEqn= 14497 Job CP sec= 4.596
Factor Done= 100% Factor Wall sec= 0.401 rate= 22.0 GFlops
Distributed sparse solver maximum pivot= 39858014.9 at node 1275 UX.
Distributed sparse solver minimum pivot= 226299.551 at node 12494 UZ.
Distributed sparse solver minimum pivot in absolute value= 226299.551
at node 12494 UZ.
DISP CONVERGENCE VALUE = 0.3397 CRITERION= 0.1699E-01
EQUIL ITER 1 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.3397
DISP CONVERGENCE VALUE = 0.3397 CRITERION= 0.1699E-01
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.3397
FORCE CONVERGENCE VALUE = 0.2063E+05 CRITERION= 1544.
EQUIL ITER 2 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.6387E-02
DISP CONVERGENCE VALUE = 0.6387E-02 CRITERION= 0.1721E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.6387E-02
FORCE CONVERGENCE VALUE = 0.1402E+05 CRITERION= 1543.
EQUIL ITER 3 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.3096E-02
DISP CONVERGENCE VALUE = 0.3096E-02 CRITERION= 0.1733E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.3096E-02
FORCE CONVERGENCE VALUE = 5753. CRITERION= 1542.
EQUIL ITER 4 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.1606E-02
DISP CONVERGENCE VALUE = 0.1606E-02 CRITERION= 0.1738E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.1606E-02
FORCE CONVERGENCE VALUE = 3513. CRITERION= 1542.
EQUIL ITER 5 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.1301E-02
DISP CONVERGENCE VALUE = 0.1301E-02 CRITERION= 0.1740E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = -0.1301E-02
FORCE CONVERGENCE VALUE = 3199. CRITERION= 1542.
EQUIL ITER 6 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.3922E-03
DISP CONVERGENCE VALUE = 0.3922E-03 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = -0.3922E-03
FORCE CONVERGENCE VALUE = 725.8 CRITERION= 1542. <<< CONVERGED
>>> SOLUTION CONVERGED AFTER EQUILIBRIUM ITERATION 6
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 80 SOLID187 0.004 0.000056
2 976 SOLID186 0.083 0.000085
3 79 SOLID187 0.004 0.000056
4 985 SOLID186 0.084 0.000086
5 10 SOLID187 0.001 0.000059
6 448 SOLID186 0.041 0.000092
7 10 SOLID187 0.001 0.000064
8 51 SOLID186 0.004 0.000087
9 4 SOLID187 0.000 0.000057
10 58 SOLID186 0.005 0.000092
11 1702 SOLID186 0.147 0.000087
12 200 CONTA174 0.012 0.000061
14 868 CONTA174 0.023 0.000026
16 115 CONTA174 0.004 0.000038
18 30 CONTA174 0.001 0.000020
20 852 CONTA174 0.015 0.000018
22 200 CONTA174 0.012 0.000062
24 98 SURF154 0.003 0.000026
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 80 SOLID187 0.001 0.000017
2 976 SOLID186 0.017 0.000017
3 79 SOLID187 0.001 0.000017
4 985 SOLID186 0.018 0.000018
5 10 SOLID187 0.000 0.000019
6 448 SOLID186 0.008 0.000019
7 10 SOLID187 0.000 0.000019
8 51 SOLID186 0.001 0.000018
9 4 SOLID187 0.000 0.000017
10 58 SOLID186 0.001 0.000021
11 1702 SOLID186 0.030 0.000018
12 200 CONTA174 0.002 0.000009
14 868 CONTA174 0.003 0.000003
16 115 CONTA174 0.001 0.000005
18 30 CONTA174 0.000 0.000003
20 852 CONTA174 0.003 0.000003
22 200 CONTA174 0.002 0.000008
24 98 SURF154 0.000 0.000003
*** LOAD STEP 1 SUBSTEP 1 COMPLETED. CUM ITER = 6
*** TIME = 0.500000 TIME INC = 0.500000
FORCE CONVERGENCE VALUE = 6425. CRITERION= 3084.
DISP CONVERGENCE VALUE = 0.8170E-03 CRITERION= 0.1741E-01 <<< CONVERGED
EQUIL ITER 1 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.8170E-03
DISP CONVERGENCE VALUE = 0.5673E-03 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.6944 SCALED MAX DOF INC = -0.5673E-03
FORCE CONVERGENCE VALUE = 1773. CRITERION= 3084. <<< CONVERGED
>>> SOLUTION CONVERGED AFTER EQUILIBRIUM ITERATION 1
*** LOAD STEP 1 SUBSTEP 2 COMPLETED. CUM ITER = 7
*** TIME = 1.00000 TIME INC = 0.500000
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
0.188 MB WRITTEN ON ELEMENT MATRIX FILE: file0.emat
7.312 MB WRITTEN ON ELEMENT SAVED DATA FILE: file0.esav
16.250 MB WRITTEN ON ASSEMBLED MATRIX FILE: file0.full
2.562 MB WRITTEN ON RESULTS FILE: file0.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
****************************************************
*************** FINISHED SOLVE FOR LS 1 *************
****************************************************
******************* SOLVE FOR LS 2 OF 4 ****************
PRINTOUT RESUMED BY /GOP
DELETE ALL SPECIFIED NODAL LOADS FROM NODE 20183 TO 20183 BY 1
NUMBER OF NODAL LOADS DELETED= 1
*** WARNING *** CP = 12.768 TIME= 06:45:47
Table _FIX is an MAPDL reserved table name. It is used to constrain
structural degrees of freedom to their current displaced status. This
table should not be used for other purposes.
SPECIFIED CONSTRAINT UX FOR SELECTED NODES 20183 TO 20183 BY 1
SET ACCORDING TO TABLE PARAMETER = _FIX
USE AUTOMATIC TIME STEPPING THIS LOAD STEP
USE 1 SUBSTEPS INITIALLY THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
FOR AUTOMATIC TIME STEPPING:
USE 10 SUBSTEPS AS A MAXIMUM
USE 1 SUBSTEPS AS A MINIMUM
TIME= 2.0000
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPPL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 12.870 TIME= 06:45:47
Material number 24 (used by element 11591) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 12.881 TIME= 06:45:47
The step data was checked and warning messages were found.
Please review output or errors file (
/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/fil
le0.err ) for these warning messages.
*** NOTE *** CP = 12.881 TIME= 06:45:47
This nonlinear analysis defaults to using the full Newton-Raphson
solution procedure. This can be modified using the NROPT command.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2025 R1 25.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 06:45:47 APR 14, 2025 CP= 13.091
--Static Structural
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 2
TIME AT END OF THE LOAD STEP. . . . . . . . . . 2.0000
AUTOMATIC TIME STEPPING . . . . . . . . . . . . ON
INITIAL NUMBER OF SUBSTEPS . . . . . . . . . 1
MAXIMUM NUMBER OF SUBSTEPS . . . . . . . . . 10
MINIMUM NUMBER OF SUBSTEPS . . . . . . . . . 1
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS. . . . 15
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . NO
TERMINATE ANALYSIS IF NOT CONVERGED . . . . . .YES (EXIT)
CONVERGENCE CONTROLS. . . . . . . . . . . . . .USE DEFAULTS
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
RSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
EPPL ALL
CONT ALL
SOLUTION MONITORING INFO IS WRITTEN TO FILE= file.mntr
>>>> PRETENSION ELEMENT STATUS <<<<
SECTION NAME PT.NODE --------STATUS-------------------- ----LOAD SOURCE----
25 20183 FIXED RELATIVE MOTION= 0.69600 D COMMAND
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS HAS BEEN MODIFIED
TO BE, NEQIT = 26, BY SOLUTION CONTROL LOGIC.
FORCE CONVERGENCE VALUE = 0.4900E+05 CRITERION= 3094.
DISP CONVERGENCE VALUE = 0.1807E-01 CRITERION= 0.1741E-01
EQUIL ITER 1 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.1807E-01
DISP CONVERGENCE VALUE = 0.1798E-01 CRITERION= 0.1741E-01
LINE SEARCH PARAMETER = 0.9947 SCALED MAX DOF INC = 0.1798E-01
FORCE CONVERGENCE VALUE = 0.3010E+05 CRITERION= 3121.
EQUIL ITER 2 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.7670E-02
DISP CONVERGENCE VALUE = 0.7173E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.9351 SCALED MAX DOF INC = 0.7173E-02
FORCE CONVERGENCE VALUE = 0.2484E+05 CRITERION= 3121.
EQUIL ITER 3 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.9770E-02
DISP CONVERGENCE VALUE = 0.9530E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.9754 SCALED MAX DOF INC = 0.9530E-02
FORCE CONVERGENCE VALUE = 0.2325E+05 CRITERION= 3127.
EQUIL ITER 4 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.8206E-02
DISP CONVERGENCE VALUE = 0.8206E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.8206E-02
FORCE CONVERGENCE VALUE = 0.1745E+05 CRITERION= 3132.
EQUIL ITER 5 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.2773E-02
DISP CONVERGENCE VALUE = 0.2661E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.9597 SCALED MAX DOF INC = 0.2661E-02
FORCE CONVERGENCE VALUE = 0.1851E+05 CRITERION= 3135.
EQUIL ITER 6 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.2297E-02
DISP CONVERGENCE VALUE = 0.2297E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.2297E-02
FORCE CONVERGENCE VALUE = 8553. CRITERION= 3137.
EQUIL ITER 7 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.2496E-02
DISP CONVERGENCE VALUE = 0.2496E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.2496E-02
FORCE CONVERGENCE VALUE = 5753. CRITERION= 3690.
EQUIL ITER 8 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.9114E-03
DISP CONVERGENCE VALUE = 0.9114E-03 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 1.000 SCALED MAX DOF INC = 0.9114E-03
FORCE CONVERGENCE VALUE = 1854. CRITERION= 3766. <<< CONVERGED
>>> SOLUTION CONVERGED AFTER EQUILIBRIUM ITERATION 8
*** LOAD STEP 2 SUBSTEP 1 COMPLETED. CUM ITER = 15
*** TIME = 2.00000 TIME INC = 1.00000
****************************************************
*************** FINISHED SOLVE FOR LS 2 *************
****************************************************
******************* SOLVE FOR LS 3 OF 4 ****************
PRINTOUT RESUMED BY /GOP
DELETE ALL SPECIFIED NODAL LOADS FROM NODE 20183 TO 20183 BY 1
NUMBER OF NODAL LOADS DELETED= 0
SPECIFIED CONSTRAINT UX FOR SELECTED NODES 20183 TO 20183 BY 1
SET ACCORDING TO TABLE PARAMETER = _FIX
USE AUTOMATIC TIME STEPPING THIS LOAD STEP
USE 1 SUBSTEPS INITIALLY THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
FOR AUTOMATIC TIME STEPPING:
USE 10 SUBSTEPS AS A MAXIMUM
USE 1 SUBSTEPS AS A MINIMUM
TIME= 3.0000
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPPL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 23.242 TIME= 06:45:56
Material number 24 (used by element 11591) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 23.258 TIME= 06:45:56
The step data was checked and warning messages were found.
Please review output or errors file (
/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/fil
le0.err ) for these warning messages.
*** NOTE *** CP = 23.258 TIME= 06:45:56
This nonlinear analysis defaults to using the full Newton-Raphson
solution procedure. This can be modified using the NROPT command.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2025 R1 25.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 06:45:56 APR 14, 2025 CP= 23.482
--Static Structural
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 3
TIME AT END OF THE LOAD STEP. . . . . . . . . . 3.0000
AUTOMATIC TIME STEPPING . . . . . . . . . . . . ON
INITIAL NUMBER OF SUBSTEPS . . . . . . . . . 1
MAXIMUM NUMBER OF SUBSTEPS . . . . . . . . . 10
MINIMUM NUMBER OF SUBSTEPS . . . . . . . . . 1
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS. . . . 15
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . NO
TERMINATE ANALYSIS IF NOT CONVERGED . . . . . .YES (EXIT)
CONVERGENCE CONTROLS. . . . . . . . . . . . . .USE DEFAULTS
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
RSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
EPPL ALL
CONT ALL
SOLUTION MONITORING INFO IS WRITTEN TO FILE= file.mntr
>>>> PRETENSION ELEMENT STATUS <<<<
SECTION NAME PT.NODE --------STATUS-------------------- ----LOAD SOURCE----
25 20183 FIXED RELATIVE MOTION= 0.69600 D COMMAND
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS HAS BEEN MODIFIED
TO BE, NEQIT = 26, BY SOLUTION CONTROL LOGIC.
FORCE CONVERGENCE VALUE = 0.4901E+05 CRITERION= 3130.
DISP CONVERGENCE VALUE = 0.8366E-01 CRITERION= 0.1741E-01
EQUIL ITER 1 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.8366E-01
DISP CONVERGENCE VALUE = 0.7799E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.9323E-01 SCALED MAX DOF INC = -0.7799E-02
FORCE CONVERGENCE VALUE = 0.2872E+06 CRITERION= 3128.
EQUIL ITER 2 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.4199E-01
DISP CONVERGENCE VALUE = 0.9193E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.2189 SCALED MAX DOF INC = -0.9193E-02
FORCE CONVERGENCE VALUE = 0.3232E+06 CRITERION= 3114.
EQUIL ITER 3 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.2300E-01
DISP CONVERGENCE VALUE = 0.6410E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.2787 SCALED MAX DOF INC = -0.6410E-02
FORCE CONVERGENCE VALUE = 0.2862E+06 CRITERION= 3104.
EQUIL ITER 4 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.1093E-01
DISP CONVERGENCE VALUE = 0.5654E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.5174 SCALED MAX DOF INC = -0.5654E-02
FORCE CONVERGENCE VALUE = 0.2004E+06 CRITERION= 3092.
EQUIL ITER 5 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.3957E-02
DISP CONVERGENCE VALUE = 0.3780E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.9553 SCALED MAX DOF INC = -0.3780E-02
FORCE CONVERGENCE VALUE = 0.2064E+05 CRITERION= 3080.
EQUIL ITER 6 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.1633E-02
DISP CONVERGENCE VALUE = 0.1391E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.8517 SCALED MAX DOF INC = 0.1391E-02
FORCE CONVERGENCE VALUE = 5975. CRITERION= 3078.
EQUIL ITER 7 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.9135E-03
DISP CONVERGENCE VALUE = 0.6763E-03 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.7404 SCALED MAX DOF INC = -0.6763E-03
FORCE CONVERGENCE VALUE = 3436. CRITERION= 3618. <<< CONVERGED
>>> SOLUTION CONVERGED AFTER EQUILIBRIUM ITERATION 7
*** LOAD STEP 3 SUBSTEP 1 COMPLETED. CUM ITER = 22
*** TIME = 3.00000 TIME INC = 1.00000
****************************************************
*************** FINISHED SOLVE FOR LS 3 *************
****************************************************
******************* SOLVE FOR LS 4 OF 4 ****************
PRINTOUT RESUMED BY /GOP
DELETE ALL SPECIFIED NODAL LOADS FROM NODE 20183 TO 20183 BY 1
NUMBER OF NODAL LOADS DELETED= 0
SPECIFIED CONSTRAINT UX FOR SELECTED NODES 20183 TO 20183 BY 1
SET ACCORDING TO TABLE PARAMETER = _FIX
USE AUTOMATIC TIME STEPPING THIS LOAD STEP
USE 1 SUBSTEPS INITIALLY THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
FOR AUTOMATIC TIME STEPPING:
USE 10 SUBSTEPS AS A MAXIMUM
USE 1 SUBSTEPS AS A MINIMUM
TIME= 4.0000
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPPL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 34.726 TIME= 06:46:07
Material number 24 (used by element 11591) should normally have at
least one MP or one TB type command associated with it. Output of
energy by material may not be available.
*** NOTE *** CP = 34.737 TIME= 06:46:07
The step data was checked and warning messages were found.
Please review output or errors file (
/tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural/fil
le0.err ) for these warning messages.
*** NOTE *** CP = 34.737 TIME= 06:46:07
This nonlinear analysis defaults to using the full Newton-Raphson
solution procedure. This can be modified using the NROPT command.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2025 R1 25.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 06:46:07 APR 14, 2025 CP= 34.928
--Static Structural
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 4
TIME AT END OF THE LOAD STEP. . . . . . . . . . 4.0000
AUTOMATIC TIME STEPPING . . . . . . . . . . . . ON
INITIAL NUMBER OF SUBSTEPS . . . . . . . . . 1
MAXIMUM NUMBER OF SUBSTEPS . . . . . . . . . 10
MINIMUM NUMBER OF SUBSTEPS . . . . . . . . . 1
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS. . . . 15
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . NO
TERMINATE ANALYSIS IF NOT CONVERGED . . . . . .YES (EXIT)
CONVERGENCE CONTROLS. . . . . . . . . . . . . .USE DEFAULTS
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
RSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
EPPL ALL
CONT ALL
SOLUTION MONITORING INFO IS WRITTEN TO FILE= file.mntr
>>>> PRETENSION ELEMENT STATUS <<<<
SECTION NAME PT.NODE --------STATUS-------------------- ----LOAD SOURCE----
25 20183 FIXED RELATIVE MOTION= 0.69600 D COMMAND
MAXIMUM NUMBER OF EQUILIBRIUM ITERATIONS HAS BEEN MODIFIED
TO BE, NEQIT = 26, BY SOLUTION CONTROL LOGIC.
FORCE CONVERGENCE VALUE = 0.4911E+05 CRITERION= 3088.
DISP CONVERGENCE VALUE = 0.1818E-01 CRITERION= 0.1741E-01
EQUIL ITER 1 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= -0.1818E-01
DISP CONVERGENCE VALUE = 0.1785E-01 CRITERION= 0.1741E-01
LINE SEARCH PARAMETER = 0.9818 SCALED MAX DOF INC = -0.1785E-01
FORCE CONVERGENCE VALUE = 0.1003E+05 CRITERION= 3067.
EQUIL ITER 2 COMPLETED. NEW TRIANG MATRIX. MAX DOF INC= 0.2201E-02
DISP CONVERGENCE VALUE = 0.2010E-02 CRITERION= 0.1741E-01 <<< CONVERGED
LINE SEARCH PARAMETER = 0.9135 SCALED MAX DOF INC = 0.2010E-02
FORCE CONVERGENCE VALUE = 2968. CRITERION= 3069. <<< CONVERGED
>>> SOLUTION CONVERGED AFTER EQUILIBRIUM ITERATION 2
*** LOAD STEP 4 SUBSTEP 1 COMPLETED. CUM ITER = 24
*** TIME = 4.00000 TIME INC = 1.00000
****************************************************
*************** FINISHED SOLVE FOR LS 4 *************
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 6.76944444
PRINTOUT RESUMED BY /GOP
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 38.596
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2025 R1 25.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 06:46:10 APR 14, 2025 CP= 38.604
--Static Structural
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 38.605 TIME= 06:46:10
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 38.609
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 6.76944444
PARAMETER _PREPTIME = 1.000000000
PARAMETER _SOLVTIME = 33.00000000
PARAMETER _POSTTIME = 0.000000000
PARAMETER _TOTALTIM = 34.00000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 1.66797753
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 0.704617897E-01
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 55197.0000
*GET _SOL_END_TIME FROM ACTI ITEM=SET TIME VALUE= 4.00000000
*IF _sol_end_time ( = 4.00000 ) EQ
4.000000 ( = 4.00000 ) THEN
/FCLEAN COMMAND REMOVING ALL LOCAL FILES
*ENDIF
--- Total number of nodes = 20313
--- Total number of elements = 9161
--- Element load balance ratio = 1.66797753
--- Time to combine distributed files = 7.046178971E-02
--- Sparse memory mode = 2
--- Number of DOF = 55197
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 9
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2025 R1 Build: 25.1 Update: UP20241202 Platform: LINUX x64
Date Run: 04/14/2025 Time: 06:46 Process ID: 3984
Operating System: Ubuntu 20.04.6 LTS
Processor Model: AMD EPYC 7763 64-Core Processor
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
AOCL-BLAS 4.2.1 Build 20240303
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 4
Number of threads per process requested : 1
Total number of cores requested : 4 (Distributed Memory Parallel)
MPI Type: OPENMPI
MPI Version: Open MPI v4.0.5
GPU Acceleration: Not Requested
Job Name: file0
Input File: dummy.dat
Core Machine Name Working Directory
-----------------------------------------------------
0 e601fcb0279a /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural
1 e601fcb0279a /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural
2 e601fcb0279a /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural
3 e601fcb0279a /tmp/ANSYS.root.1/AnsysMechB88C/Project_Mech_Files/StaticStructural
Latency time from master to core 1 = 2.018 microseconds
Latency time from master to core 2 = 1.977 microseconds
Latency time from master to core 3 = 2.005 microseconds
Communication speed from master to core 1 = 16484.95 MB/sec
Communication speed from master to core 2 = 17445.53 MB/sec
Communication speed from master to core 3 = 14709.64 MB/sec
Total CPU time for main thread : 33.7 seconds
Total CPU time summed for all threads : 38.8 seconds
Elapsed time spent obtaining a license : 0.5 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.2 seconds
Elapsed time spent solution - preprocessing : 0.8 seconds
Elapsed time spent computing solution : 32.3 seconds
Elapsed time spent solution - postprocessing : 0.1 seconds
Elapsed time spent post-processing model (/POST1) : 0.0 seconds
Equation solver used : Sparse (symmetric)
Equation solver computational rate : 87.3 Gflops
Equation solver effective I/O rate : 200.7 GB/sec
Sum of disk space used on all processes : 190.7 MB
Sum of memory used on all processes : 740.0 MB
Sum of memory allocated on all processes : 3326.0 MB
Physical memory available : 31 GB
Total amount of I/O written to disk : 2.1 GB
Total amount of I/O read from disk : 11.4 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2025 R1 Build 25.1 UP20241202 LINUX x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Max Database Used(Master) 20 MB Max Scratch Used(Master) 189 MB |
| Max Database Used(Workers) 1 MB Max Scratch Used(Workers) 216 MB |
| Sum Database Used(All) 23 MB Sum Scratch Used(All) 717 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 38.772 Time = 06:46:10 |
| Elapsed Time (sec) = 35.000 Date = 04/14/2025 |
| |
*-----------------------------------------------------------------------------*
Close mechanical#
Close the mechanical instance.
mechanical.exit()
Total running time of the script: (0 minutes 50.271 seconds)