Note
Go to the end to download the full example code.
Capture images after a solve#
Using supplied files, this example shows how to resume a MECHDAT file and capture the images of all results in a folder on the disk.
Download required files#
Download the required files. Print the file paths for the MECHDAT file and script files.
import os
from ansys.mechanical.core import launch_mechanical
from ansys.mechanical.core.examples import download_file
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
mechdat_path = download_file(
"example_03_simple_bolt_new.mechdat", "pymechanical", "00_basic"
)
print(f"Downloaded the MECHDAT file to: {mechdat_path}")
script_file_path = download_file(
"example_02_capture_images_helper.py", "pymechanical", "00_basic"
)
print(f"Downloaded the script files to: {script_file_path}")
Downloaded the MECHDAT file to: /home/runner/.local/share/ansys_mechanical_core/examples/example_03_simple_bolt_new.mechdat
Downloaded the script files to: /home/runner/.local/share/ansys_mechanical_core/examples/example_02_capture_images_helper.py
Launch Mechanical#
Launch a new Mechanical session in batch, setting the cleanup_on_exit
argument to False
. To close this Mechanical session when finished,
this example must call the mechanical.exit()
method.
mechanical = launch_mechanical(batch=True, cleanup_on_exit=False)
print(mechanical)
Ansys Mechanical [Ansys Mechanical Enterprise]
Product Version:251
Software build date: 11/27/2024 09:34:44
Initialize the variable needed for opening the MECHDAT file#
Set the mechdat_path
variable for later use.
Make the variable compatible for Windows, Linux, and Docker containers.
project_directory = mechanical.project_directory
print(f"project directory = {project_directory}")
# Upload the file to the project directory.
mechanical.upload(file_name=mechdat_path, file_location_destination=project_directory)
# Build the path relative to the project directory.
base_name = os.path.basename(mechdat_path)
combined_path = os.path.join(project_directory, base_name)
mechdat_path_modified = combined_path.replace("\\", "\\\\")
mechanical.run_python_script(f"mechdat_path='{mechdat_path_modified}'")
# Verify the path for the MECHDAT file.
result = mechanical.run_python_script(f"mechdat_path")
print(f"MECHDATA file is stored on the server at: {result}")
project directory = /tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/
Uploading example_03_simple_bolt_new.mechdat to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/.: 0%| | 0.00/7.06M [00:00<?, ?B/s]
Uploading example_03_simple_bolt_new.mechdat to dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/.: 100%|██████████| 7.06M/7.06M [00:00<00:00, 325MB/s]
MECHDATA file is stored on the server at: /tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/example_03_simple_bolt_new.mechdat
Open the MECHDAT file#
Run the script to open the MECHDAT file.
mechanical.run_python_script("ExtAPI.DataModel.Project.Open(mechdat_path)")
''
Initialize the variable needed for the image directory#
Set the image_dir
variable for later use.
Make the variable compatible for Windows, Linux, and Docker containers.
# Open the MECHDAT file to change the project directory.
project_directory = mechanical.project_directory
print(f"project directory = {project_directory}")
image_directory_modified = project_directory.replace("\\", "\\\\")
mechanical.run_python_script(f"image_dir='{image_directory_modified}'")
# Verify the path for image directory.
result_image_dir_server = mechanical.run_python_script(f"image_dir")
print(f"Images are stored on the server at: {result_image_dir_server}")
project directory = /tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/example_03_simple_bolt_new_Mech_Files/
Images are stored on the server at: /tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/example_03_simple_bolt_new_Mech_Files/
Run the mechanical script#
Run the Mechanical script file for creating the images.
mechanical.run_python_script_from_file(script_file_path)
''
Download the image and plot#
Download one image file from the server to the current working directory and plot using matplotlib.
def get_image_path(image_name):
return result_image_dir_server + image_name
def display_image(path):
print(f"Printing {path} using matplotlib")
image1 = mpimg.imread(path)
plt.figure(figsize=(15, 15))
plt.axis("off")
plt.imshow(image1)
plt.show()
image_name = "Total Deformation @ 1 sec_Right.png"
image_path_server = get_image_path(image_name)
if image_path_server != "":
current_working_directory = os.getcwd()
local_file_path_list = mechanical.download(
image_path_server, target_dir=current_working_directory
)
image_local_path = local_file_path_list[0]
print(f"Local image path : {image_local_path}")
display_image(image_local_path)
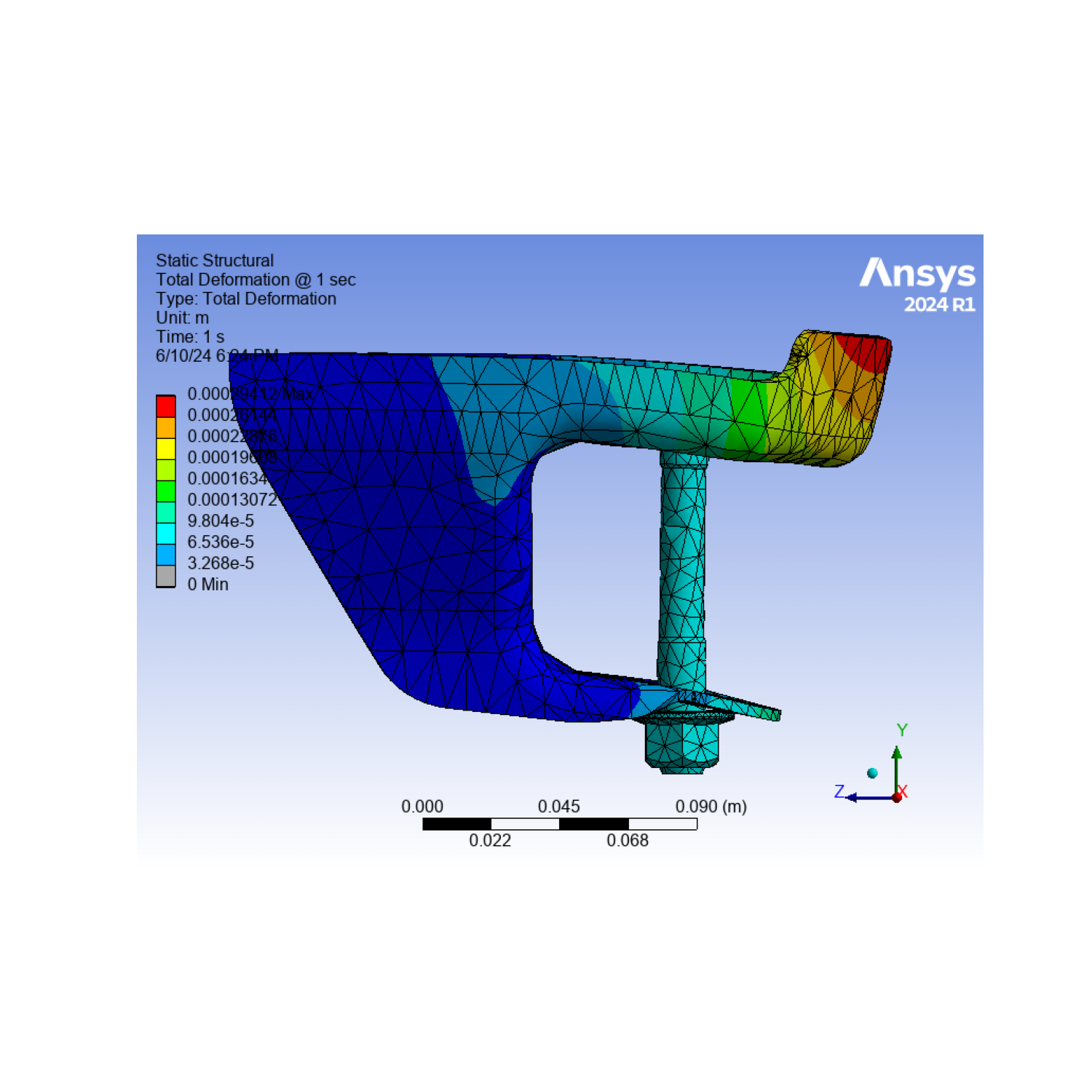
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/example_03_simple_bolt_new_Mech_Files/Total Deformation @ 1 sec_Right.png to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/00_basic/Total Deformation @ 1 sec_Right.png: 0%| | 0.00/117k [00:00<?, ?B/s]
Downloading dns:///127.0.0.1:10000:/tmp/ANSYS.root.1/AnsysMech4880/Project_Mech_Files/example_03_simple_bolt_new_Mech_Files/Total Deformation @ 1 sec_Right.png to /home/runner/work/pymechanical-examples/pymechanical-examples/examples/00_basic/Total Deformation @ 1 sec_Right.png: 100%|██████████| 117k/117k [00:00<00:00, 549MB/s]
Local image path : /home/runner/work/pymechanical-examples/pymechanical-examples/examples/00_basic/Total Deformation @ 1 sec_Right.png
Printing /home/runner/work/pymechanical-examples/pymechanical-examples/examples/00_basic/Total Deformation @ 1 sec_Right.png using matplotlib
Clear the data#
Clear the data so it isn’t saved to the project.
mechanical.clear()
Close Mechanical#
Close the Mechanical instance.
mechanical.exit()
Total running time of the script: (0 minutes 32.429 seconds)